GPTsのアクション機能を使う事でGPTsからAPIにファイルを送信したり、APIで作ったファイルをGPTsに返すこともできます。GPTsでファイルを扱う方法を解説します。
GPTsアクションのファイル送受信の仕組み
GPTsからファイルを送る
アクションから直接ファイルをAPIに送る事はできません。アクションはプロンプトから添付されたファイルを一度サーバーに格納し、5分間のみ有効なダウンロードリンクを作成します。APIではダウンロードリンクからファイルを取得して使用します。
GPTsにファイルを返す
アクションに返すファイルをbase64エンコーディングして所定のjsonにして返すことでGPTsにファイルのダウンロードリンクが作成されます。こちらも5分間のみ有効です。
仕様
いずれも名前が重要です。名前が間違っていると添付ファイルを認識しなかったり、ファイルをダウンロードできません。
GPTsからファイルを送る際のパラメータ
以下の4つの項目を持つobjectの配列をopenaiFileIdRefs
という名前で定義します。
- id: GPTsアクションが付ける識別子
- name: ファイル名
- mime_type: ファイルのMIMEタイプ
- download_link: 5分間有効なダウンロードリンクのURL
GPTsにファイルを返す際のResponse
以下の3つの項目を持つobjectの配列をopenaiFileResponse
という名前で定義します。
- name: ファイル名
- mime_type: ファイルのMIMEタイプ
- content: base64エンコードされたファイルの内容
アクションのスキーマ設定
GPTsからファイルを送る際のスキーマ設定
"paths" : {
"/send" : {
"post" : {
"operationId": "Send files.",
"description" : "Uploads a file reference using its file id.",
"requestBody" : {
"content" : {
"application/json" : {
"schema" : {
"$ref" : "#/components/schemas/openaiFileIdRefs"
}
}
},
"required" : true
},
"responses" : {
"200" : {
"description" : "200 response",
"content" : {
"application/json" : {
"schema" : {
"$ref" : "#/components/schemas/Empty"
}
}
}
}
}
}
}
},
"components" : {
"schemas" : {
"Empty" : {
"title" : "Empty Schema",
"type" : "object",
"properties" : { }
},
"openaiFileIdRefs" : {
"title" : "openaiFileIdRefs",
"type" : "object",
"properties" : {
"openaiFileIdRefs" : {
"type" : "array",
"items" : {
"required" : [ "download_link", "id", "mime_type", "name" ],
"type" : "object",
"properties" : {
"name" : {
"type" : "string",
"description" : "The name of the file"
},
"id" : {
"type" : "string",
"description" : "The unique identifier of the file"
},
"mime_type" : {
"type" : "string",
"description" : "The MIME type of the file"
},
"download_link" : {
"type" : "string",
"description" : "The download link for the file"
}
}
}
}
}
}
}
}
GPTsにファイルを返す際のスキーマ設定
"paths" : {
"/download" : {
"get" : {
"operationId": "Download files",
"description" : "Download files with the reception ID",
"parameters" : [ {
"name" : "id",
"in" : "query",
"required" : true,
"schema" : {
"type" : "string"
}
} ],
"responses" : {
"200" : {
"description" : "200 response",
"content" : {
"application/json" : {
"schema" : {
"$ref" : "#/components/schemas/openaiFileResponse"
}
}
}
}
}
}
}
},
"components" : {
"schemas" : {
"openaiFileResponse" : {
"title" : "openaiFileResponse",
"type" : "object",
"properties" : {
"openaiFileResponse" : {
"type" : "array",
"items" : {
"required" : [ "content", "mime_type", "name" ],
"type" : "object",
"properties" : {
"name" : {
"type" : "string",
"description" : "The name of the file. This will be visible to the user."
},
"mime_type" : {
"type" : "string",
"description" : "The MIME type of the file. This is used to determine eligibility and which features have access to the file."
},
"content" : {
"type" : "string",
"description" : "The base64 encoded contents of the file.",
"format" : "byte"
}
}
}
}
}
}
}
}
APIの処理
GPTsアクションから送られたファイルを取得するコード
download_linkからファイルを取得するのにrequestsライブラリを使用しています。
import json
import requests
def lambda_handler(event, context):
# パラメータを取得
body = json.loads(event['body'])
name = body["openaiFileIdRefs"][0].get('name')
id = body["openaiFileIdRefs"][0].get('id')
mime_type = body["openaiFileIdRefs"][0].get('mime_type')
download_link = body["openaiFileIdRefs"][0].get('download_link')
# download_linkからファイルをダウンロード
response = requests.get(download_link)
GPTsアクションにファイルを返す処理
S3から取得したファイルをGPTsアクションに返すサンプルです。
import json
import boto3
import base64
def lambda_handler(event, context):
# S3からファイルを取得する
s3 = boto3.client('s3')
response = s3.get_object(Bucket='bucket_name', Key='key')
body = response['Body'].read().decode('utf-8')
files = []
files.append({
'name': "formatted.txt",
'mime_type': 'text/plain',
'content': base64.b64encode(body.encode('utf-8')).decode('utf-8')
})
openaiFileResponse = {
'openaiFileResponse': files
}
return {
'statusCode': 200,
'body': json.dumps(openaiFileResponse),
'headers': {
'Content-Type': 'application/json'
}
}
動作確認
GPTsアクションからのファイル送信
正しく動くと下記の画像のようにAPIに送信されたファイルを確認出来ます。
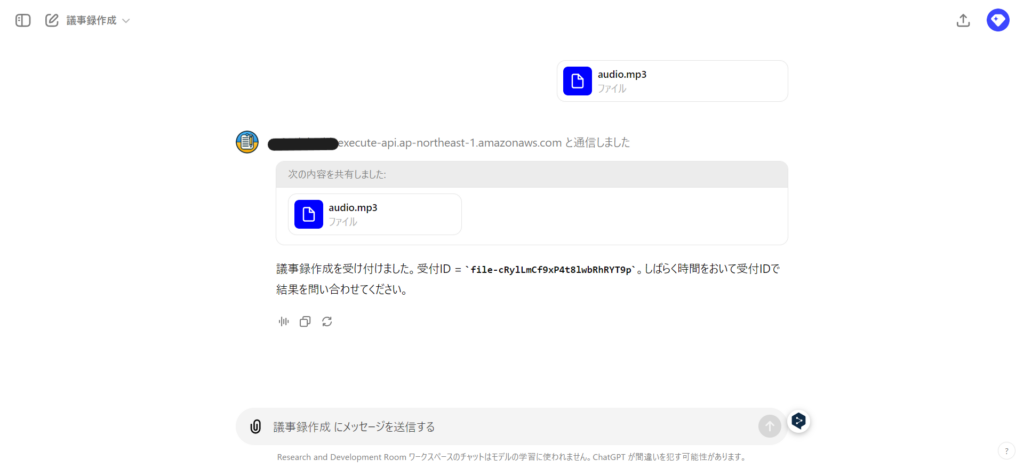
GPTsアクションへのファイルを返す
APIから正しくファイルを返すことができると下記の画像のようにファイルをダウンロードできます。
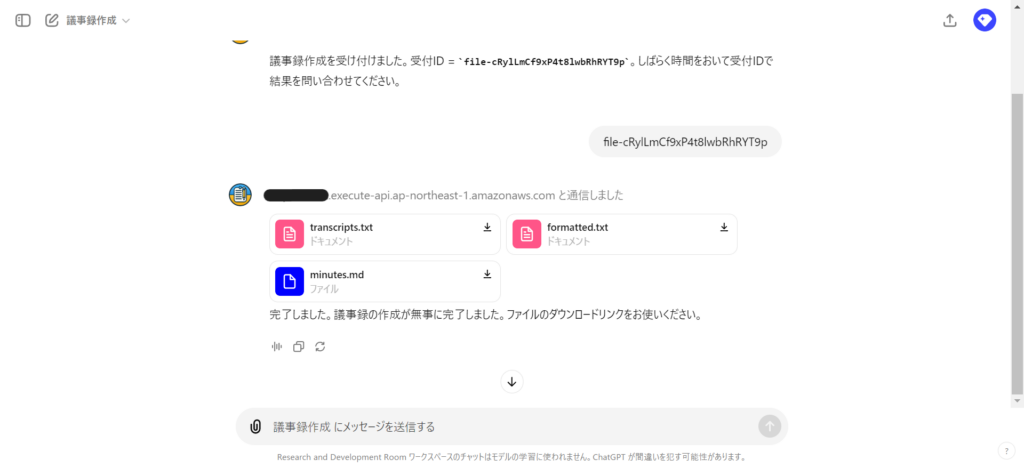
注意点
GPTsアクションのタイムアウト
GPTsアクションのタイムアウトは45秒です。私が作ったAPIではdownload_linkからファイルを取得して処理するには時間がかかりすぎてタイムアウトしてしまいました。サイズの大きいファイルを扱う場合はファイルを非同期で処理するような仕組みが必要かもしれません。私の場合はdownload_linkだけ受け取って、結果を受付IDで問い合わせられるようにしました。
まとめ
長くなってしまいましたが、GPTsアクションでファイルを送受信する方法を解説しました。GPTsでファイルを送受信できるとやれることの幅が広がります。この記事を参考にGPTsアクションでファイルの送受信に挑戦してみて下さい。
この記事を書いた人
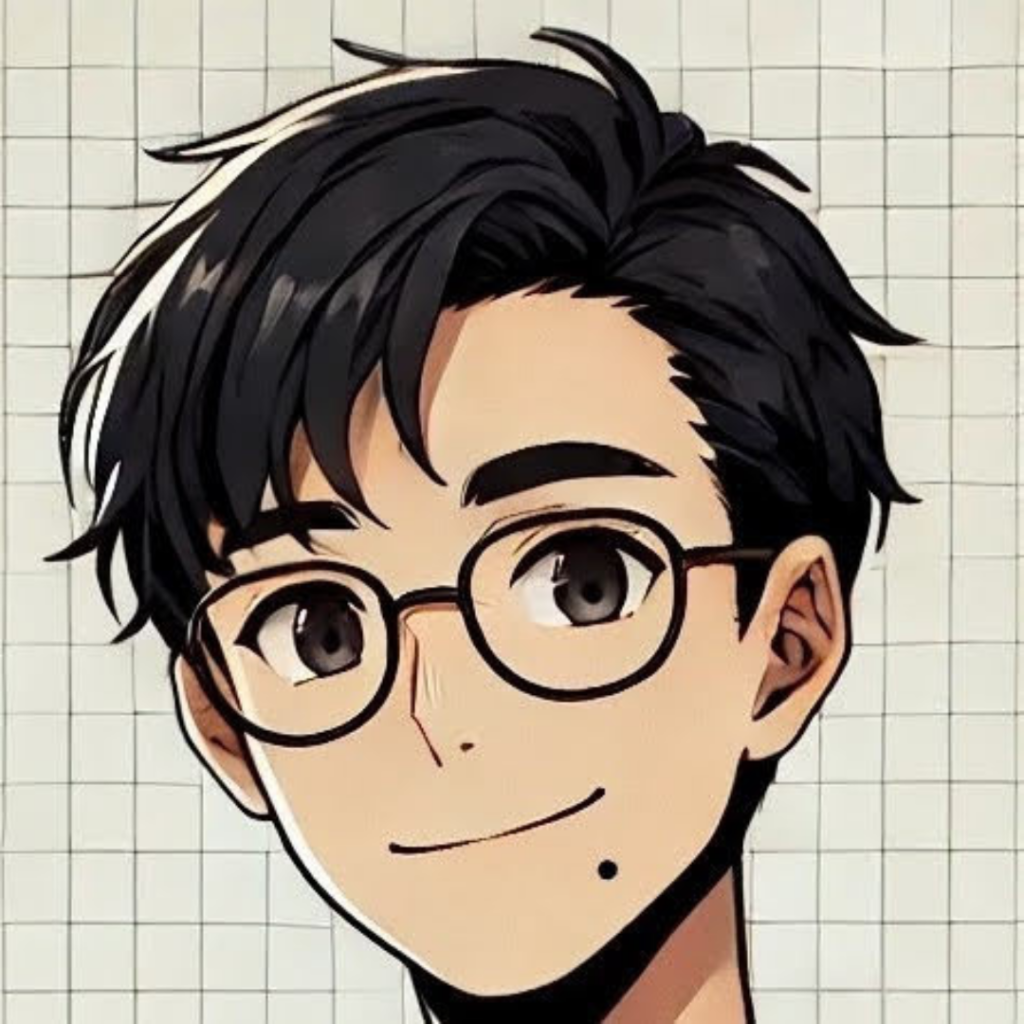